Linux
Shell
The command line interface is called a shell and on Ubuntu, the default shell is bash.
After logging in, you will be in your HOME directory. Usually, it is in '/home/username' but on this cluster, your home will be inside '/home_nfs/username'.
This directory is stored on the storage array of the head node of the cluster and its content will be shared over the network.
Whichever server you log in, you will always get into your home folder (represented by a ~ in the prompt).
Here is the meaning behind the prompt look :
[demo@datamaster ~]$ (1) [user@hostname directory]$ (2)
1 | You are the user demo and you are in your home directory on the datamaster server. |
2 | User@Server directory |
Windows |
Linux |
|
Root |
c:\ |
/ |
User’s directory |
C:\Users\username |
/home_nfs/username |
Programs path |
c:\Program Files\ |
/usr/bin/ |
How to move in the filesystem tree
Here is an example that shows how to move in the filesystem tree :
[demo@datamaster ~]$ pwd
/home_nfs/demo
[demo@datamaster ~]$ mkdir scripts
[demo@datamaster ~]$ cd scripts
[demo@datamaster scripts]$ pwd
/home_nfs/demo/scripts
[demo@datamaster scripts]$ cd ..
[demo@datamaster ~]$ pwd
/home_nfs/demo/
[demo@datamaster ~]$ mkdir -p sources/python
[demo@datamaster ~]$ cd /home_nfs/demo/sources/python
[demo@datamaster python]$ pwd
/home_nfs/demo/sources/python
Return to your home |
cd |
cd ~ |
Go up in the tree |
cd .. |
|
Return to last dir |
cd - |
|
Move to a relative path |
cd ../../usr/local/share |
|
Move to an absolute path |
cd /usr/local/share |
Creating files and directories
You can create files in several ways.
The touch command, which is used to modify the timestamps of a file, also allows you to create an empty file if no file with the name passed as argument exists in the current folder.
$ touch hello.txt
$ ls hello.txt -l
-rw-rw-r--. 1 demo demo 0 26 jun 11:50 hello.txt
This file can be opened with a test editor like nano or vim.
$ nano hello.txt (1)
$ cat hello.txt
Hello World !
...
$ ls hello.txt -l
-rw-rw-r--. 1 demo demo 342 26 jun 11:50 hello.txt
1 | See capture below. |
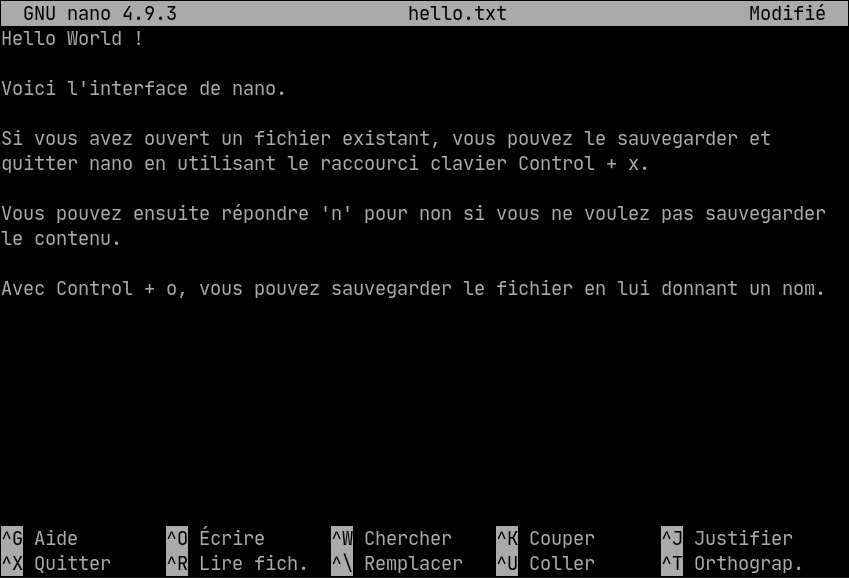
A file can be created by redirecting a stream :
$ ls /usr/lib/ -l >> apps.txt
$ ls -lh apps.txt
-rw-rw-r--. 1 guttadauriaa guttadauriaa 44K 26 jun 12:07 apps.txt
A double >> adds the stream to the end of an existing file but a single one > will replace the existing content by the new one. |
Erasing files and folders
The rm command allows you to delete files.
$ rm hello.txt
$ ls hello.txt -l
-rw-rw-r--. 1 demo demo 0 26 jun 11:50 hello.txt
The rmdir command allows you to delete empty directories.
$ mkdir files/
$ touch files/hello.txt
$ ls hello.txt -l
-rw-rw-r--. 1 demo demo 0 26 jun 11:50 hello.txt
$ rmdir files
rmdir: cannot delete 'files': The directory is not empty
$ rm files/*.txt
$ rmdir files
Programs and Environment Variables
In POSIX systems like Linux, applications are mainly installed in the /usr/bin directory for users and /usr/sbin for system commands. Applications can share code with dynamic libraries which are stored in /usr/lib or /usr/lib64.
You can run a program by entering its full path in a terminal :
$ /usr/bin/g++ --version
g++ (Ubuntu 9.4.0-1ubuntu1~20.04.1) 9.4.0
For not having to enter full paths to applications, a variable named PATH is defined. It contains a list of directories where are stored the system’s apps.
Thanks to it, you can run these apps by entering their names :
$ echo $PATH
/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:/usr/games:/usr/local/games:/snap/bin
$ gcc --version
gcc (Ubuntu 9.4.0-1ubuntu1~20.04.1) 9.4.0
$ which gcc
/usr/bin/gcc
This works out of the box for the apps installed from the software manager of the system but not for the programs you downloaded or built in your home directory.
For these, you will need to add their directories in the PATH :
# Downloading Go language
$ wget https://dl.google.com/go/go1.18.6.linux-amd64.tar.gz
$ tar -xf go1.18.6.linux-amd64.tar.gz -C ~/bin/
# Adding its directory in the PATH
$ export PATH=$HOME/bin/go/bin:$PATH
/home_nfs/demo/bin/go/bin:/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:/usr/games:/usr/local/games:/snap/bin
$ go version
go version go1.18.6 linux/amd64
$ which go
/home_nfs/demo/bin/go/bin/go
The modified PATH variable will only be active for the current session and will revert after exiting. In order to preserve this change, you can add this line in your ~/.bashrc ou ~/.bash_profile file.
$ echo 'export PATH=$HOME/bin/go/bin:$PATH' >> ~/.bashrc
This use case is really simple but often software needs dynamic libraries (.so on Linux, .dll on Windows). Then, you need to add their folders to another variable named $LD_LIBRARY_PATH. Furthermore, other environment variables could be necessary at execution or when compiling a framework.
Then comes another problem. What happens when, for example, you need to use different versions of OpenMPI for different projects ? Of course, you can add several directories leading to the mpirun command in the PATH variable but only the first occurence would be used.
Modifying theses variables can be tedious and are sources of errors. This is the reason why the environment modules have been created.
Scripts
A script is a file containing a set of instructions in an interpreted language like bash, Python or Ruby as examples.
Here is a bash script that write "Hello World !" on the standard output :
#!/bin/bash
NAME=$1
if [[ -n $NAME ]]; then
echo "Hello $NAME !"
exit
fi
echo "Hello World !"
[demo@datamaster ~]$ ls scripts
my_script.sh
[demo@datamaster ~]$ ./scripts/my_script.sh Adriano (1)
Hello Adriano !
[demo@datamaster ~]$ cd scripts
[demo@datamaster scripts]$ ./my_script.sh (1)
Hello World !
[demo@datamaster scripts]$ cd -
[demo@datamaster ~]$ /home_nfs/demo/scripts/my_script.sh (2)
Hello World !
1 | Called from a relative path with an argument |
2 | Called from an absolute path (from the root '/' of the tree) |
The script need to be executable in order to call it directly.
Here is how you can verify if it has the execution permission :
[demo@datamaster scripts]$ ls -l script.sh
-rw-rw-r--. 1 demo demo 104 29 jun 15:05 script.sh
[demo@datamaster scripts]$ chmod u+x script.sh
[demo@datamaster scripts]$ ls -l script.sh
-rwxrw-r--. 1 demo demo 104 29 jun 15:05 script.sh
Here is a list of commands
- ls
-
List files
- cd
-
Change directory : move around the file systems
- pwd
-
Present working directory : print where you are.
- mkdir
-
Make directory : Create a new directory
- rm
-
Remove file : Delete a file
- rmdir
-
Remove directory : delete an empty directory
- file
-
Give information on a file
- nano
-
edit a file (simple)
- vim
-
edit a file (advanced)
- ssh
-
Secure Shell : go to another server through an encrypted connection
- htop
-
Show computer status (quit with 'q')
- chmod
-
Change modificator : change permissions on a file or directory
- chown
-
Change owner : change the owner or group owner of a file
- man
-
Manual : display the manual of a command